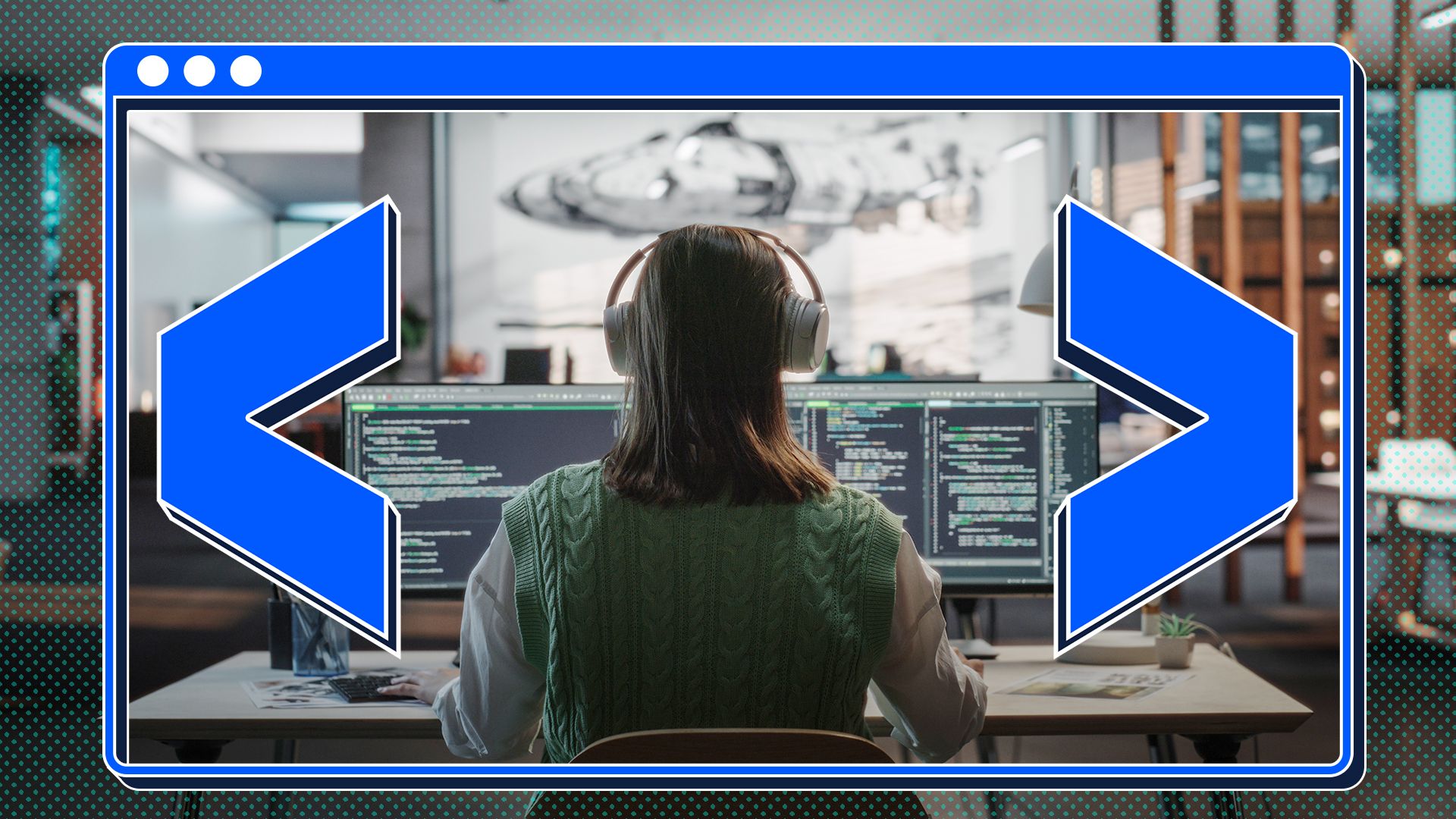
Are you a beginner programmer looking to go to the next level? Here are some of the best habits I adopted and how they made me not only a better programmer but also a better professional.
7
Start With a Strong Foundation
When I first started programming, I fell into the trap of rushing the basics and jumping straight to the more juicy stuff like frameworks and advanced tools. I wanted to build cool apps and shiny websites right away. So, I tried to skip the boring stuff as much as possible.
However, at some point, I realized something. I could copy-paste code and get it to work. But it was all spaghetti code, jumbled together, barely working without any optimization. I couldn’t solve any problems on my own. Ultimately, I was forced to revisit the basics again and again.
One way to build this foundation is to challenge yourself with simple problems before diving into bigger projects. Platforms like HackerRank and CSES are great for this. Another essential part of building a foundation is learning one programming language deeply before branching out.
6
Do More Thinking Than Writing Code
Whenever you want to build something, you probably open the code editor and start writing code. We do this so that we can quickly see a tangible result. However, in my experience, I’ve seen that this often leads to messy, incomplete, or sometimes entirely wrong solutions.
A problem I faced a lot was when I was halfway in, I realized that I didn’t take one case or another into account when coding the solution. I had to start over. If I had an overall high-level solution before writing the code, this wouldn’t have happened.
Programming isn’t just about writing code. It’s about crafting a solution to a problem, often under some constraints. Like any complex problem, the best solution comes from clear, deliberate thinking. This is from one of my favorite books, The Pragmatic Programmer:
In order to be a Pragmatic Programmer, we’re challenging you to think about what you’re doing while you’re doing it…Never run on auto-pilot. Constantly be thinking, critiquing your work in real time.
One thing that has made me a better problem-solver is writing pseudocode before writing the actual code. For example, when designing a complex algorithm, I’ll sketch a rough logical plan in plain English.
5
Learn From the Official Documentation
When I first started programming, I avoided official documentation like the plague. It looked overwhelming, full of jargon, and honestly, a little boring. I preferred sticking to tutorials and YouTube videos. But as I started working on real-world projects, I realized that tutorials only scratched the surface. If I wanted to fully understand a language, framework, or tool, the official documentation was my best resource.
Official documentation is a user manual written by the people who created the language or tool you’re learning. Tutorials tend to focus on specific use cases, but documentation shows you the full capabilities of a technology, including features you may not have known existed.
For example, when I first learned Python, I relied heavily on tutorials to understand the basics. But when I started using libraries like pandas, I found myself needing more than just examples. The pandas documentation became my lifeline.
4
Write Clean Code
There’s a running joke in the programming community. If your program runs, don’t touch it. It’s just a joke, though.
When I was getting started on programming, I tried competitive programming. Though I was enjoying it, I adopted many bad habits from it. In most cases, making the program work was the only thing that mattered. If the code ran without errors, I considered it a success, even if it was a tangled mess of variables and magic numbers. But as I started working on more real-world projects, I realized how crucial clean code is.
Clean code makes your programs easy to read, debug, and maintain. There are many best practices, principles, and conventions that go into this. For example, descriptive names for variables and functions, good documentation, consistent coding style, and more. Let’s look at an example of ugly code.
def pro(s, x): i = 0
for k in range(len(s)):
i += s[k] * x[k]
return i
It works. But can you make anything out of it? Probably not. Now have a look at the cleaner version:
def calculate_dot_product(vector_a, vector_b): dot_product = 0
for index in range(len(vector_a)):
dot_product += vector_a[index] * vector_b[index]
return dot_product
This version is much more meaningful. That’s how writing clean code can fully transform your projects.
3
Develop Strong Debugging Skills
No matter how much experience you gain as a programmer, debugging is an inevitable part of the process. I’ve spent countless hours tracking down sneaky bugs that seemed to hide in plain sight. At first, debugging felt frustrating. Over time, I learned to approach it strategically.
The first step in debugging is to slow down and observe. I used to rush into my code, blindly changing lines, hoping something would work. It rarely did. Instead, start by asking questions. What is the program supposed to do? What is it doing? Where does behavior diverge?
There are several tools and techniques that make debugging less daunting. The print()
statement is often the first line of defense. Another useful technique is rubber duck debugging, where you explain your code, step by step, to an inanimate object or a person. Often, simply articulating the problem out loud reveals the solution. I’ve solved bugs just by explaining them to myself.
2
Network With Other Programmers
When I first started programming, I thought of it as a solo journey. While programming often involves solitary focus, the moments where I grew the most came from connecting with other programmers.
I’ve joined many programming Facebook groups, Discord servers, and subreddits (check out r/learnprogramming.) When I’m facing problems, I can reach out for help. In my free time, I try to contribute to the community as well, so that beginners can get help just like I used to.
I once landed a programming gig solely through networking. It was one of the biggest projects I’ve worked on, and I learned a lot in the process. I’ve also met great programmers by attending hackathons and learned new things and strategies from them.
1
Build Something That Solves a Real-Life Problem
One of the most rewarding aspects of programming is the ability to turn ideas into tools that make life easier. You can watch tutorials or read books endlessly, but nothing compares to the learning you gain when you tackle a real-world problem. That’s when the theories, syntax, and algorithms truly come alive.
You can find real-life projects in many ways. What problems do you face? Can you solve them by building an app? Ask your friends and relatives about their problems. You can also go to GitHub and find open-source projects.
When I started learning Java backend using Spring Boot, I decided to make an agricultural system to automate many of the tasks. I learned about many things that I wouldn’t have from tutorials.
When you’re new to programming, it’s easy to fall into traps and make mistakes. By adopting great habits and learning from experience, you can have a head start. That’ll make you a better programmer.
Source link